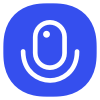
Sign up to save your podcasts
Or
In this lesson, we're going to do an overview of functions. This will be just a general discussion to lay out a framework for understanding how functions work and how we can use them.
More specifically, we'll be talking about:
In an earlier lesson, we said functions are like the verbs of a programming language. If you want to get something done, it's probably going to involve a function.
Function CallsThe Arduino programming language is essentially the same as C++ and a lot like C. So, it’s chock-full of different types of functions that do all kinds of things for us. If you can think of something, there's probably a function that does it.
To use this grand variety of functions, all we have to do is type the name of the specific function that we want to use. That's it. You just type its name.
Then you follow its name by an opening and closing parenthesis. Sometimes, you have to put values in between those parentheses.
Let's take this function called digitalWrite, for example. The digitalWrite function wants to know a pin number on your Arduino, and then it wants to know a state, either HIGH or LOW.
Once you give it that information, it will apply either five volts, which would be a HIGH state, or zero volts, which would be a LOW state.
When we type digitalWrite in our program, that is called a function call. I want to make sure that you’ve heard that terminology, function call, because you’ll be hearing it quite a bit. We'll actually be going over a lot of terminology in this lesson.
Function ParametersSome functions require information from us in order to work properly. The function digitalWrite needed to know two things. It needed to know pin number and high or low voltage.
The pieces of information that the function needs to operate are called the function's parameters.
You might be wondering, “How am I supposed to know what the parameters of a function are?” That's actually a really good question because when you look at the word digitalWrite, there's nothing in the name that alludes to what parameters it needs.
In order to know what the parameters are, you have to look it up in a resource called the reference. The reference is like a user manual for programming languages.
For functions that you use all the time, you'll become very familiar with what the parameters are. You won't have to look it up all the time.
However, for functions that are unfamiliar, you'll probably have to refer to the reference frequently. It's really easy to use, and you'll learn a lot more about it throughout the course.
Passing ArgumentsBack to our discussion on parameters. As said before, parameters are what a function wants or expects.
The actual values that you give to the function, though, are called the arguments. In our digiWrite example, we have the number three and the state as HIGH.
Those specific values are the arguments. This might sound like a case of semantics to you, but it's actually quite different.
Parameters are just the general definition of what the function expects, like pin number or state. The argument is the specific value that you pass to a function, like 3 or HIGH.
One more term to learn is “passing”. When I give a function arguments, it's called passing. I pass the arguments to a function.
Okay, so let's do a quick recap. When we use a function, it's called a function call because we're calling a function. Seems straightforward, right?
The information a function needs to operate are called its parameters. The actual values we send into the function are called arguments. Lastly, when we send those arguments, we say we’re passing the arguments to a function.
Phew, that's a lot of terminology! Keep in mind that that's all it really is. It's just fancy computer programming words.
The reason I want to talk about it here is because we're going to be using these terms throughout the course, and I'm sure you'll hear them elsewhere. Therefore, I think if you understand these terms, it will help us communicate better since we'll all be speaking the same language.
Different Ways Functions WorkNow let's move on to the different ways that functions work. For some functions, like our example digitalWrite, do something for us. We might pass the number 13 and HIGH to digitalWrite, and digitalWrite then applies five volts at pin 13. The function actually does something physical for us.
Other functions just return values. For example, the function digitalRead monitors a pin on the Arduino and lets you know the state of the pin, either HIGH or LOW. It only has one parameter, which is the number of the pin that you want to check.
To make sense of this, let's look at this line of code as an example. We have pinState = digitalRead(3). This line of code assigns the output of the digitalRead function to the variable pinState.
So when this gets executed, the function digitalRead gets called, and we pass it the value three. Therefore, three is our argument.
Now, digitalRead goes and checks the state of pin three. It's either going to be HIGH or LOW. Then, it sends that value back to our program.
When it sends that value back, it's called returning the value. In other words, the function digitalRead returns the state of that pin. The value that gets returned will then be assigned to the variable pinState.
Another example would be a Celsius to Fahrenheit converter function. It takes a number in Celsius and then returns the different, converted value in Fahrenheit. If you want that new Fahrenheit value, you need to assign it to a variable.
Alright, so far we've been talking about functions that take parameters. In order for them to work, you have to give them some information. However, there are other functions that don't need any information to work.
One common example is the millis function. When you call the millis function, it pulls the number of milliseconds that the current Arduino sketch has been running. Millis uses hardware that's integral to the integrated circuit the Arduino uses. It's going to pull information behind-the-scenes and return this value to you. You don't have to do anything.
There are other functions that do this, as well. You don't have to give it any information. It goes out, it does stuff, and it gives that information back to you. So in this line of code, the variable timing will be assigned the number of milliseconds that the millis function returns.
Finally, there are some functions that don't take parameters and don't return values. They just do something when they're called.
Maybe you're working on a project that waters plants at different time intervals. You might need a function that simply opens a valve. In this line of code, we have OpenValve. The OpenValve function doesn't take any parameters. It simply opens a valve that we specify elsewhere.
When we use a function, it's important to realize that there's more than meets the eye. For example, do you know what DNA stands for? It stands for deoxyribonucleic acid. It's quite a mouthful to say.
So instead of saying deoxyribonucleic acid, people just shorten it and use the acronym DNA. It's a quick and easy way to communicate deoxyribonucleic acid without actually having to say deoxyribonucleic acid.
Functions are similar to this in that they allow quick and easy access to a mouthful of useful code - the function definition. For every function name that you use, like digitalWrite or digitalRead, there's a function definition that gets executed for you behind the scenes.
The function definition spells out exactly how that function is going to behave, what parameters it will use, whether or not it will return any values, and the like.
In most cases, you're going to care very little about the function definition. You're going to be more interested in how to use the function. After all, the power of a function is its ability to reduce your workload.
Programming languages are filled with tons of useful functions. Some are used far more frequently than others. You'll find that you'll get quite familiar with a couple dozen or so of your most frequently used, most effective functions.
Other functions you'll slowly integrate into your knowledge. Later in the course, you're even going to learn how to write your own function definitions so that you can make your own handy user-defined functions, but that's going to be for a later time.
ReviewAlright, let's review this lesson. We discussed what it means to make a function call. We also discussed that parameters are the general values that a function expects in order to work.
The specific values we give a function are the arguments. Giving the function these arguments is called passing the arguments to the function.
Finally, we talked about different ways that functions work. Some take arguments and then physically do something. Some take arguments and then return a value. Some don't take any arguments and but still return a value. Some don't take any arguments and don't return a value, but they still do something.
We also touched on a lot about terminology in this lesson. Again, the goal of this lesson was just to layout a framework. I wanted to give you an overview of how we're going to be interacting with all different types of functions throughout the course. I look forward to seeing you in the next lesson.
3.8
2222 ratings
In this lesson, we're going to do an overview of functions. This will be just a general discussion to lay out a framework for understanding how functions work and how we can use them.
More specifically, we'll be talking about:
In an earlier lesson, we said functions are like the verbs of a programming language. If you want to get something done, it's probably going to involve a function.
Function CallsThe Arduino programming language is essentially the same as C++ and a lot like C. So, it’s chock-full of different types of functions that do all kinds of things for us. If you can think of something, there's probably a function that does it.
To use this grand variety of functions, all we have to do is type the name of the specific function that we want to use. That's it. You just type its name.
Then you follow its name by an opening and closing parenthesis. Sometimes, you have to put values in between those parentheses.
Let's take this function called digitalWrite, for example. The digitalWrite function wants to know a pin number on your Arduino, and then it wants to know a state, either HIGH or LOW.
Once you give it that information, it will apply either five volts, which would be a HIGH state, or zero volts, which would be a LOW state.
When we type digitalWrite in our program, that is called a function call. I want to make sure that you’ve heard that terminology, function call, because you’ll be hearing it quite a bit. We'll actually be going over a lot of terminology in this lesson.
Function ParametersSome functions require information from us in order to work properly. The function digitalWrite needed to know two things. It needed to know pin number and high or low voltage.
The pieces of information that the function needs to operate are called the function's parameters.
You might be wondering, “How am I supposed to know what the parameters of a function are?” That's actually a really good question because when you look at the word digitalWrite, there's nothing in the name that alludes to what parameters it needs.
In order to know what the parameters are, you have to look it up in a resource called the reference. The reference is like a user manual for programming languages.
For functions that you use all the time, you'll become very familiar with what the parameters are. You won't have to look it up all the time.
However, for functions that are unfamiliar, you'll probably have to refer to the reference frequently. It's really easy to use, and you'll learn a lot more about it throughout the course.
Passing ArgumentsBack to our discussion on parameters. As said before, parameters are what a function wants or expects.
The actual values that you give to the function, though, are called the arguments. In our digiWrite example, we have the number three and the state as HIGH.
Those specific values are the arguments. This might sound like a case of semantics to you, but it's actually quite different.
Parameters are just the general definition of what the function expects, like pin number or state. The argument is the specific value that you pass to a function, like 3 or HIGH.
One more term to learn is “passing”. When I give a function arguments, it's called passing. I pass the arguments to a function.
Okay, so let's do a quick recap. When we use a function, it's called a function call because we're calling a function. Seems straightforward, right?
The information a function needs to operate are called its parameters. The actual values we send into the function are called arguments. Lastly, when we send those arguments, we say we’re passing the arguments to a function.
Phew, that's a lot of terminology! Keep in mind that that's all it really is. It's just fancy computer programming words.
The reason I want to talk about it here is because we're going to be using these terms throughout the course, and I'm sure you'll hear them elsewhere. Therefore, I think if you understand these terms, it will help us communicate better since we'll all be speaking the same language.
Different Ways Functions WorkNow let's move on to the different ways that functions work. For some functions, like our example digitalWrite, do something for us. We might pass the number 13 and HIGH to digitalWrite, and digitalWrite then applies five volts at pin 13. The function actually does something physical for us.
Other functions just return values. For example, the function digitalRead monitors a pin on the Arduino and lets you know the state of the pin, either HIGH or LOW. It only has one parameter, which is the number of the pin that you want to check.
To make sense of this, let's look at this line of code as an example. We have pinState = digitalRead(3). This line of code assigns the output of the digitalRead function to the variable pinState.
So when this gets executed, the function digitalRead gets called, and we pass it the value three. Therefore, three is our argument.
Now, digitalRead goes and checks the state of pin three. It's either going to be HIGH or LOW. Then, it sends that value back to our program.
When it sends that value back, it's called returning the value. In other words, the function digitalRead returns the state of that pin. The value that gets returned will then be assigned to the variable pinState.
Another example would be a Celsius to Fahrenheit converter function. It takes a number in Celsius and then returns the different, converted value in Fahrenheit. If you want that new Fahrenheit value, you need to assign it to a variable.
Alright, so far we've been talking about functions that take parameters. In order for them to work, you have to give them some information. However, there are other functions that don't need any information to work.
One common example is the millis function. When you call the millis function, it pulls the number of milliseconds that the current Arduino sketch has been running. Millis uses hardware that's integral to the integrated circuit the Arduino uses. It's going to pull information behind-the-scenes and return this value to you. You don't have to do anything.
There are other functions that do this, as well. You don't have to give it any information. It goes out, it does stuff, and it gives that information back to you. So in this line of code, the variable timing will be assigned the number of milliseconds that the millis function returns.
Finally, there are some functions that don't take parameters and don't return values. They just do something when they're called.
Maybe you're working on a project that waters plants at different time intervals. You might need a function that simply opens a valve. In this line of code, we have OpenValve. The OpenValve function doesn't take any parameters. It simply opens a valve that we specify elsewhere.
When we use a function, it's important to realize that there's more than meets the eye. For example, do you know what DNA stands for? It stands for deoxyribonucleic acid. It's quite a mouthful to say.
So instead of saying deoxyribonucleic acid, people just shorten it and use the acronym DNA. It's a quick and easy way to communicate deoxyribonucleic acid without actually having to say deoxyribonucleic acid.
Functions are similar to this in that they allow quick and easy access to a mouthful of useful code - the function definition. For every function name that you use, like digitalWrite or digitalRead, there's a function definition that gets executed for you behind the scenes.
The function definition spells out exactly how that function is going to behave, what parameters it will use, whether or not it will return any values, and the like.
In most cases, you're going to care very little about the function definition. You're going to be more interested in how to use the function. After all, the power of a function is its ability to reduce your workload.
Programming languages are filled with tons of useful functions. Some are used far more frequently than others. You'll find that you'll get quite familiar with a couple dozen or so of your most frequently used, most effective functions.
Other functions you'll slowly integrate into your knowledge. Later in the course, you're even going to learn how to write your own function definitions so that you can make your own handy user-defined functions, but that's going to be for a later time.
ReviewAlright, let's review this lesson. We discussed what it means to make a function call. We also discussed that parameters are the general values that a function expects in order to work.
The specific values we give a function are the arguments. Giving the function these arguments is called passing the arguments to the function.
Finally, we talked about different ways that functions work. Some take arguments and then physically do something. Some take arguments and then return a value. Some don't take any arguments and but still return a value. Some don't take any arguments and don't return a value, but they still do something.
We also touched on a lot about terminology in this lesson. Again, the goal of this lesson was just to layout a framework. I wanted to give you an overview of how we're going to be interacting with all different types of functions throughout the course. I look forward to seeing you in the next lesson.
191 Listeners
28,375 Listeners
1 Listeners